JavaScript
https://en.wikipedia.org/wiki/JavaScriptJavaScript
JavaScript is a versatile, high-level, interpreted (or just-in-time compiled) programming language that is one of the core technologies of the web.
Here's a comprehensive overview:
History and Background
Birth of JavaScript:
JavaScript was created in 10 days in May 1995 by Brendan Eich, while he was an engineer at Netscape. Originally named Mocha, it was later renamed to LiveScript, and finally to JavaScript when it was deployed in the Netscape Navigator 2.0 browser.
Evolution:
Over the years, JavaScript has evolved significantly. The need for standardization led to the birth of ECMAScript (ES), of which JavaScript is an implementation. ECMAScript versions like ES3 (1999), ES5 (2009), and ES6 (2015, also known as ES2015) brought significant changes and improvements.
Features:
Interpreted Language:
JavaScript code is typically executed line by line, on-the-fly, without a need for compilation.
Dynamic Typing:
Variables in JavaScript can hold any type of data, and their type can be changed at any time.
Object-Oriented & Functional:
It supports both object-oriented and functional programming paradigms.
First-Class Functions:
Functions are first-class objects, meaning they can be passed around as arguments, returned from other functions, and assigned to variables.
Prototype-Based:
Instead of the classical inheritance seen in languages like Java or C++, JavaScript uses prototype-based inheritance.
Event-Driven:
Well-suited for event-driven applications like web pages.
Concurrency & the Event Loop:
JavaScript is single-threaded but uses events and callbacks to handle concurrent operations without multi-threading, utilizing the Event Loop and the Web APIs provided by browsers.
Key Concepts:
Variables:
Let (block-scoped), const (block-scoped & constant), and var (function-scoped).
Data Types:
Primitive: Undefined, Null, Boolean, Number, String, BigInt, and Symbol.
Object: Arrays, Functions, Dates, and more.
Functions:
Can be declared traditionally or as arrow functions.
Objects & Prototypes:
Objects can be created using literal syntax, constructors, or Object.create(). Inheritance is achieved through prototypes.
Asynchronous Programming:
Callbacks, Promises, and Async/Await are ways to handle asynchronous operations.
Closures:
Functions that capture and remember references to variables from their containing scope.
The 'this' Keyword:
Refers to an object, the context in which a function is called.
Modules:
ES6 introduced a module system to organize and structure code.
Environment:
Browsers:
JavaScript runs natively in web browsers. Each browser has its own JavaScript engine, e.g., V8 in Chrome.
Node.js:
A runtime that allows JavaScript to be run server-side. It's built on Chrome's V8 engine.
Mobile Apps, Desktop Apps:
Frameworks like React Native or Electron enable JavaScript to be used outside the web.
Modern Development & Ecosystem:
Libraries & Frameworks:
jQuery, React, Angular, Vue.js, and many more.
Package Managers:
npm (Node Package Manager) and yarn are popular tools to manage dependencies.
Transpilers & Bundlers:
Babel allows you to use next-gen JavaScript by converting it into older versions. Webpack, Rollup, and Parcel are popular bundlers.
Development Tools:
ESLint for linting, Jest for testing, and many others.
Advancements in JavaScript:
ECMAScript Proposals:
The TC39 committee continually proposes new features and updates to the ECMAScript specification. Once a proposal reaches Stage 4, it gets added to the next yearly ECMAScript release.
WebAssembly (Wasm):
Although not strictly JavaScript, Wasm is an important milestone for web development. It allows high-performance execution of code from languages like C and C++ in the browser, working alongside JavaScript.
Common Uses of JavaScript:
Web Development:
JavaScript plays a fundamental role in dynamic web page creation, enabling interactive content.
Backend Development:
With Node.js, JavaScript can be used to build scalable server-side applications.
Mobile App Development:
Frameworks like React Native or Ionic enable developers to write mobile applications using JavaScript.
Desktop Applications:
Electron and similar frameworks allow developers to create cross-platform desktop applications using JavaScript, HTML, and CSS.
Game Development:
With engines like Phaser or Three.js, developers can build browser-based games.
IoT (Internet of Things):
With platforms like Johnny-Five, JavaScript can be used to control hardware devices.
Challenges & Criticisms:
Performance:
Native languages (like C or Java) generally outperform JavaScript in CPU-intensive scenarios.
Callback Hell:
Asynchronous JavaScript, especially with older callback patterns, can lead to nested callbacks, making code harder to read and maintain. This has been somewhat alleviated with Promises and async/await.
Global Scope:
Earlier versions of JavaScript had issues with the global scope and potential for variable clashes. This has been mitigated with ES6 modules and tools like IIFEs (Immediately Invoked Function Expressions).
Diversity of Frameworks:
The rapid evolution and vast number of libraries and frameworks can be overwhelming for newcomers.
Security:
Cross-Site Scripting (XSS):
Attackers might inject malicious scripts to be run on a user's browser.
Cross-Site Request Forgery (CSRF):
Attackers may trick a user into performing actions they did not intend.
JavaScript's versatility and wide adoption make it an indispensable language for modern development. Despite its challenges, the language's adaptability, and the community's willingness to innovate, ensure that JavaScript will remain relevant for many years to come. Whether you're a beginner just starting out or an experienced developer, there's always something new to learn and explore in the world of JavaScript.

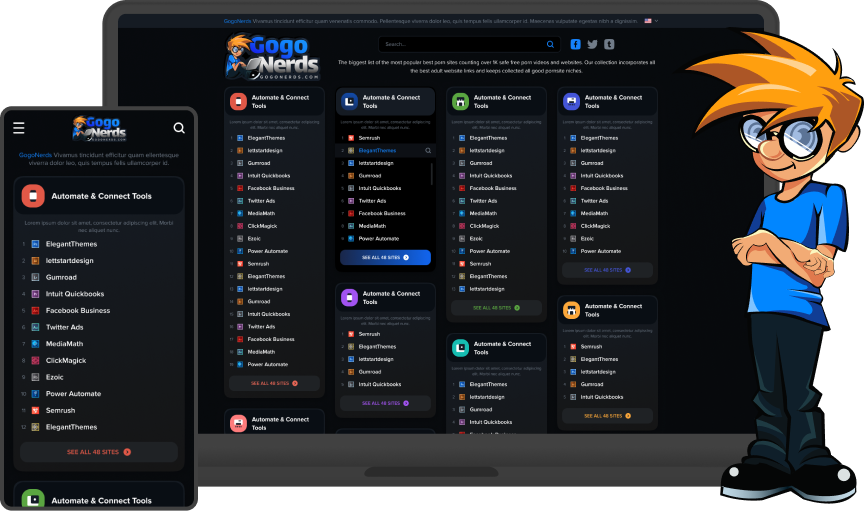