C++ (C Plus Plus)
https://isocpp.org/C++ (C Plus Plus)
C++ is a versatile, general-purpose programming language that has been around for several decades. Here's a detailed overview of C++:
History:
Origin: C++ was developed by Bjarne Stroustrup at Bell Labs starting in 1979, as an enhancement to the C programming language.
Name: Originally named "C with Classes", it was later renamed C++ in 1983. The "++" is the increment operator in C, indicating that C++ is an evolution of C.
Key Features:
Object-Oriented: C++ introduced classes, inheritance, polymorphism, and encapsulation, making it object-oriented. This allows for better organization and structure in programs, especially as they grow larger.
Low-Level Access: Just like C, C++ provides pointers which offer direct memory management capabilities.
Standard Template Library (STL): This is a library of template classes and functions, which provides collections (like vectors, lists, and maps) and algorithms (like sort, find).
Performance: As a compiled language, C++ code can be optimized to run extremely fast, which is one reason it's frequently used in performance-critical applications.
Portability: While not as portable as C due to variations in compilers and libraries, well-written C++ can be compiled and run on many different platforms.
Syntax and Semantics:
C++ retains much of C's syntax, but introduces new keywords and symbols to support object-oriented and generic programming features.
Major Concepts:
Classes and Objects: The foundation of object-oriented programming. Classes define blueprints for objects, and objects are instances of those classes.
Inheritance: Allows a class (child) to inherit attributes and methods from another class (parent).
Polymorphism: Allows objects of different classes to be treated as objects of a common super class. The most common use is through the use of virtual functions in base classes that are overridden in derived classes.
Encapsulation: The bundling of data and methods that operate on that data into a single unit or class.
Templates: Allows functions and classes to operate on generic types. Useful for creating data structures and algorithms that can work on any data type.
RAII (Resource Acquisition Is Initialization): A programming principle which ensures that resources (like memory and file handles) are acquired during object creation and released during object destruction.
Exception Handling: C++ introduces try, catch, and throw keywords to handle exceptions in a structured manner.
Standard Library:
C++ comes with a rich set of libraries, known as the C++ Standard Library.
The STL (Standard Template Library) is a part of this, providing containers like vector, list, map, etc., and algorithms like sort(), find(), etc.
The library also provides utilities for strings, streams (for I/O operations), date/time, etc.
Development and Standardization:
C++ has gone through multiple standard revisions: C++98, C++03, C++11, C++14, C++17, and C++20 (as of my last update in September 2021).
Each revision added new features, libraries, and improvements. For instance, C++11 introduced lambda functions, range-based for loops, and smart pointers (std::shared_ptr, std::unique_ptr).
Applications:
C++ is used in various domains: system/software development, game development, real-time simulations, embedded systems, high-performance server and client applications, and much more.
Tools:
There are various IDEs and compilers available for C++ development, such as GCC (GNU Compiler Collection), Clang, Microsoft's Visual Studio, and more.
Learning C++:
Due to its depth and breadth, learning C++ can be challenging but is rewarding. There are many resources available, including books, online tutorials, and courses. It's often recommended to learn C before diving into C++ for a smoother learning curve.
Advanced C++ Concepts:
Move Semantics: Introduced in C++11, it allows resources (like memory) to be efficiently moved from one object to another. This is accomplished using rvalue references and the standard library functions std::move() and std::forward().
Lambda Expressions: Also introduced in C++11, they allow the creation of anonymous function objects. They're especially useful in conjunction with STL algorithms.
Concurrency: C++11 introduced the <thread>, <mutex>, and <future> headers, enabling multi-threading and concurrency support directly in the language.
Attributes: Provide a standardized way to specify compiler behavior. For example, [[nodiscard]] and [[maybe_unused]] introduced in C++17.
Concepts: Introduced in C++20, they specify template constraints in a more readable manner compared to traditional SFINAE (Substitution Failure Is Not An Error).
Modules: A long-awaited feature that finally arrived with C++20, aiming to replace header files and reduce compilation times.
Modern C++ Guidelines:
Prefer Automatic Lifetime Management: Use smart pointers (std::unique_ptr, std::shared_ptr) rather than raw pointers to manage memory.
Use Auto Type Deduction: Especially when the type is verbose or redundant.

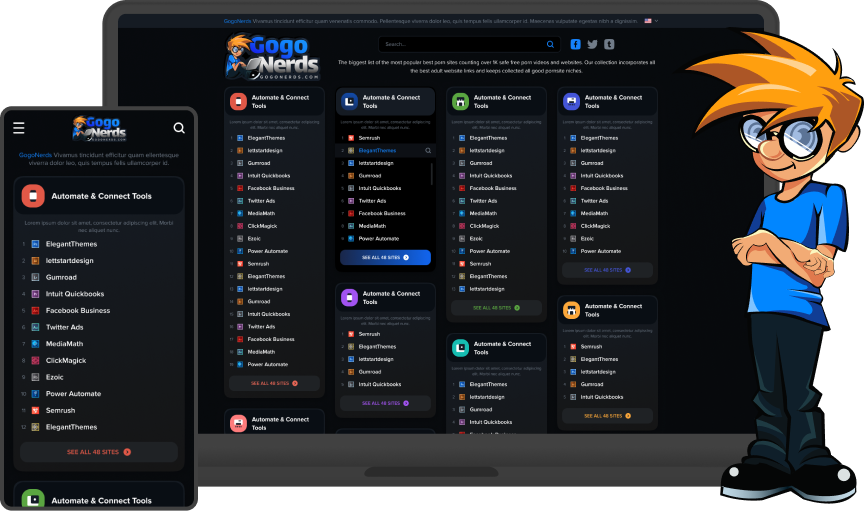