C# (C Sharp)
https://learn.microsoft.com/en-us/dotnet/csharp/C# (C Sharp)
C# (pronounced "C sharp") is a modern, object-oriented programming language developed by Microsoft as part of its .NET initiative. Since its introduction in the early 2000s, C# has evolved into a versatile language used for a wide array of applications, including desktop software, web services, mobile apps, and game development. Its design emphasizes simplicity, robustness, and developer productivity, making it a popular choice among programmers.
History and development:
C# was developed in the late 1990s and early 2000s, primarily by Anders Hejlsberg, who is also known for creating Turbo Pascal and leading the design of Delphi. Microsoft announced C# and the .NET Framework in July 2000 as part of its strategy to compete with Sun Microsystems' Java platform.
The language was submitted to the European Computer Manufacturers Association (ECMA) and later to the International Organization for Standardization (ISO) for standardization, ensuring its openness and cross-platform capabilities. Over the years, C# has undergone significant evolution, with major releases introducing new features that keep it modern and competitive.
Key features
Object-oriented programming (OOP)
C# is inherently object oriented, supporting the four main pillars of OOP:
Encapsulation
Bundling data with methods that operate on that data.
Inheritance
Creating new classes from existing classes to promote code reuse.
Polymorphism
Allowing entities to be represented in multiple forms.
Abstraction
Simplifying complex reality by modeling classes appropriate to the problem.
Type Safety
C# is a statically typed language, meaning that type checking occurs at compile time. This reduces runtime errors and enhances code reliability. Features such as nullable types and type inference (var keywords) provide flexibility while maintaining type safety.
Automatic Memory Management
C# uses a garbage collector to manage memory allocation and deallocation automatically. This reduces memory leaks and pointer errors common in languages such as C++ and allows developers to focus on application logic rather than memory management.
Rich Standard Library
The .NET framework provides an extensive class library, offering prebuilt classes for common tasks such as file I/O, networking, and data structures. This accelerates development by reducing the need to write boilerplate code.
Language Integrated Query (LINQ)
Introduced in C# 3.0, LINQ allows developers to write queries directly within C#, providing a consistent model for working with data across various sources, such as collections, XML, and databases.
Asynchronous Programming
C# supports asynchronous programming via the async and await keywords, simplifying the development of applications that perform time-consuming operations without freezing the user interface.
Cross-platform Development
With the introduction of the .NET Core (now known as .NET 5 and above), C# has become truly cross-platform, enabling developers to build applications that run on Windows, macOS, and Linux.
Syntax
Basic structure
A simple C# program:
Variables and Data Types
C# supports various data types, including integers (int), floating-point numbers (float, double), booleans (bool), characters (char), and strings (string). The variables are declared with a type followed by a name:
Control Flow Statements
C# provides standard control flow constructs:Conditional Statements: if, otherwise if, else, switchLoops: for, foreach, while do-whileExample:
Methods
Methods are defined within classes and contain code to perform actions:
Classes and Objects
Classes are blueprints for objects. They contain fields (variables) and methods (functions):
Creating an object:
Inheritance and polymorphism
Inheritance allows a class to inherit members from a base class:
Polymorphism is achieved via virtual and override keywords:
Interfaces
Interfaces define contracts that implementing classes must fulfill:
Delegates and Events
Delegates are type-safe pointers to methods, and events are used with delegates for publish–subscribe patterns:
Generics
Generics allow the creation of classes and methods with a placeholder for the type:
Advanced features
Language Integrated Query (LINQ)
LINQ enables the query of collections via an SQL-like syntax:
Asynchronous Programming
Asynchronous methods improve application responsiveness:
Dynamic Language Features
C# supports dynamic typing via the dynamic keyword, allowing for more flexible code at the cost of compile-time type checking:
Functional Programming Constructs
C# incorporates functional programming elements:
Lambda expressions:
Pattern Matching:
Immutable Data Structures: Using readonly and immutable collections.
Ecosystem and Tools
.NET Framework and.NET Core
Originally, C# was tied to the .NET Framework, which was Windows-only. With the advent of the .NET Core (which is now unified under .NET 5 and later versions), C# applications can run across platforms.
Integrated development environments (IDEs)
Visual Studio
A comprehensive IDE with features such as IntelliSense, debugging, and designer tools.
Visual Studio Code
A lightweight, cross-platform code editor with C# support via extensions.
Rider
A cross-platform .NET IDE by JetBrains.
Package Management with NuGet
NuGet is the package manager for .NET, allowing developers to share and consume reusable code.
Testing Frameworks
MSTest: Microsoft's testing framework.
NUnit: A popular open-source unit testing framework.
xUnit.net: A modern testing framework for all .NET languages.
Applications of C#
Desktop Applications
Using frameworks such as Windows Presentation Foundation (WPF) and Windows Forms, developers can create rich desktop applications for Windows.
Web development
ASP. NET Core: A cross-platform framework for building web applications and services.
Blazor: Allows running C# code in the browser via WebAssembly.
Mobile development
Xamarin: Enables cross-platform mobile app development for iOS and Android via C#.
MAUI (multiplatform app UI): The evolution of Xamarin. Forms allow developers to create native user interfaces.
Game development
Unity Engine: One of the most popular game engines uses C# for scripting.
Godot Engine: Supports C# via Mono.
Cloud Services
C# is extensively used in developing cloud services and applications, especially with Microsoft Azure.
Community and Support
C# has a vibrant community and extensive documentation. Resources include:
Microsoft Docs: Official documentation and tutorials.
GitHub: Many open-source projects and the Roslyn compiler are hosted here.
Stack Overflow: A platform for asking and answering programming questions.

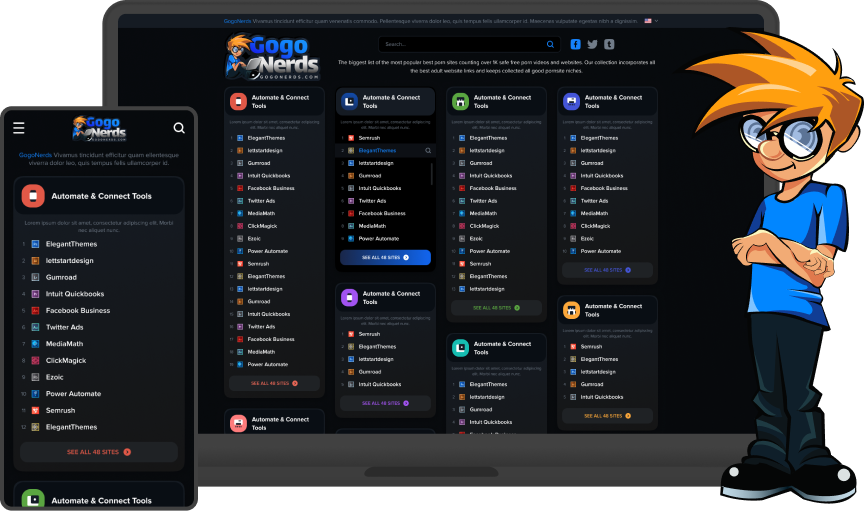